AWS SAM CLI lets you test serverless apps on your computer before deploying to AWS. Here's what you need to know:
- Install AWS SAM CLI, AWS CLI, Python, and Docker
- Create a new SAM project and understand the template file
- Test Lambda functions, API Gateway, and event-driven apps locally
- Use advanced features like custom runtimes, Step Functions, and Lambda layers
Key benefits of local testing:
- Find and fix issues quickly
- Save time and money on cloud testing
- Test thoroughly before AWS deployment
Feature | Description |
---|---|
Local running | Test functions without AWS account |
Debugging | Use breakpoints to find issues |
Testing | Check app functionality locally |
Deployment | Push tested app to AWS |
Follow best practices like organizing code, handling environment variables, and simulating AWS services for effective local testing. Common issues include permissions, dependencies, and Docker-related problems, but solutions are available.
Related video from YouTube
AWS SAM CLI basics
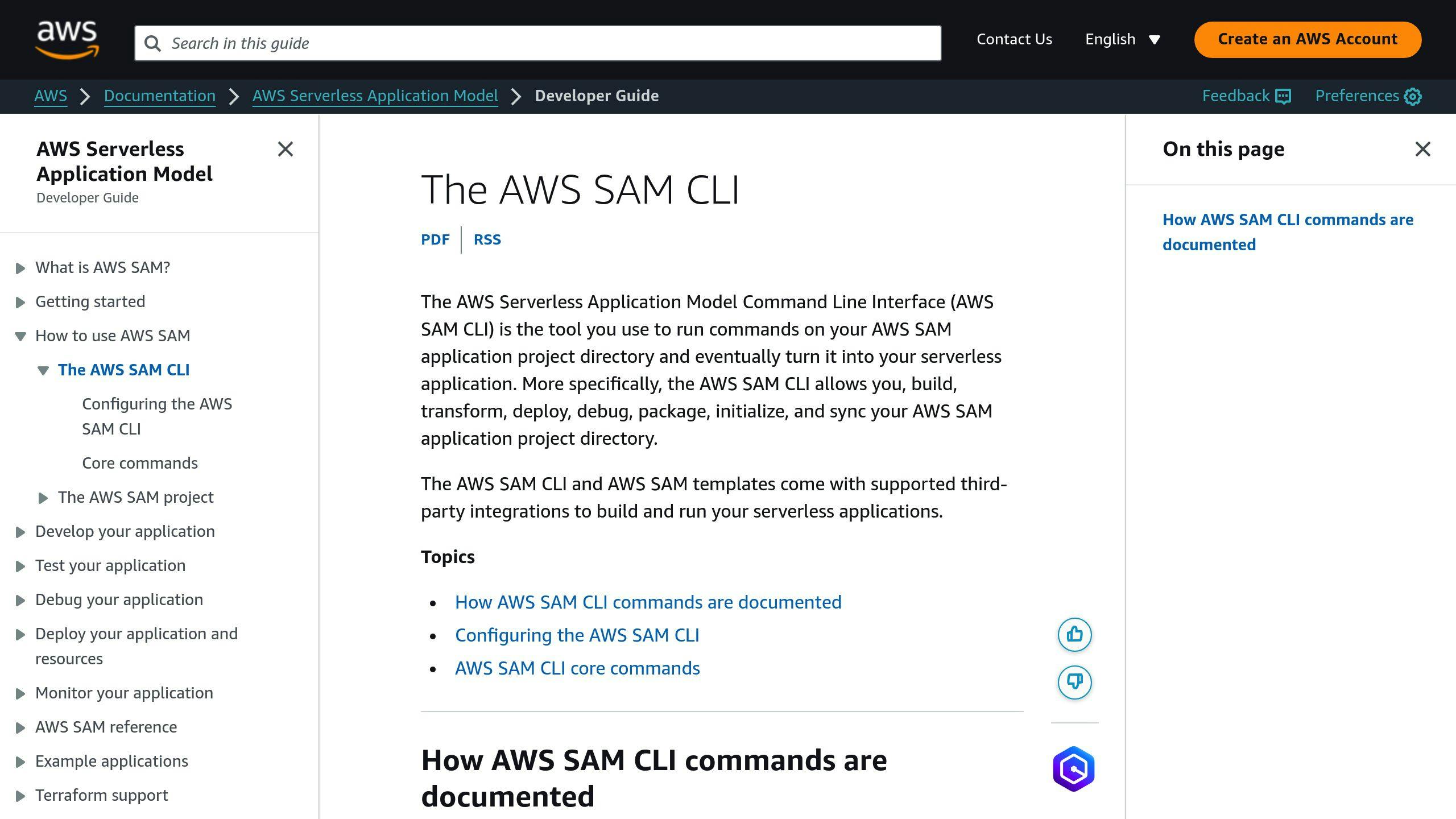
What is AWS SAM CLI?
AWS SAM CLI is a tool for building, testing, and deploying serverless apps on AWS. It works with the AWS SAM framework to help developers create and manage serverless apps. With SAM CLI, you can work on your apps locally before putting them on AWS.
SAM CLI lets you:
- Make new serverless projects
- Build and package apps
- Run functions on your computer
- Test and fix bugs in your apps
- Put your apps on AWS
Main features of AWS SAM CLI
Here are the key things SAM CLI can do:
Feature | Description |
---|---|
Local running | Use serverless functions on your computer without an AWS account |
Debugging | Find and fix problems in your code with tools like breakpoints |
Testing | Check if your app works correctly using built-in testing features |
Deployment | Put your app on AWS using SAM CLI |
How AWS SAM CLI helps with local testing
SAM CLI makes local testing better in these ways:
- Faster: Test your apps quickly without using AWS
- Cheaper: Save money by not using AWS resources for testing
- Easier: Work on your app without needing an AWS account
- More accurate: Test your app in a setup that's close to the real thing
By using SAM CLI for local testing, you can:
- Try out new ideas quickly
- Find and fix problems in your code
- Make sure your app works well before putting it on AWS
Before you start
Setting up an AWS account
To use AWS SAM CLI, you need an AWS account. Here's how to create one:
- Visit AWS website
- Click "Create an AWS account"
- Fill out the form
- Verify your email
- Provide payment info
Installing AWS CLI

AWS CLI lets you manage AWS services from your computer. To install:
Operating System | Installation Steps |
---|---|
Windows | 1. Download installer from AWS website 2. Run installer |
macOS (Homebrew) | Run: brew install awscli |
Linux | Run: sudo apt-get install awscli |
After installing, run aws configure
to set up your account.
Setting up Python

AWS SAM CLI needs Python. To set it up:
- Download Python from Python website
- Install Python 3.6 or later
- Check installation: Run
python --version
Installing Docker

Docker helps run serverless apps locally. To install:
Operating System | Installation Steps |
---|---|
Windows/macOS | 1. Download installer from Docker website 2. Run installer |
Linux | Run: sudo apt-get install docker.io |
Check installation: Run docker --version
Installing AWS SAM CLI
Installation steps for Windows, macOS, and Linux
Here's how to install AWS SAM CLI on different operating systems:
Operating System | Installation Steps |
---|---|
Windows | 1. Download the MSI installer from AWS SAM CLI GitHub releases 2. Run the installer 3. Follow the prompts |
macOS (Homebrew) | Run these commands: brew tap aws/tap brew install aws-sam-cli |
Linux (Ubuntu) | Run these commands: sudo apt-get update sudo apt-get install aws-sam-cli |
After installing, open a new command prompt or terminal window.
Checking your installation
To make sure AWS SAM CLI is working:
- Open your terminal or command prompt
- Type this command:
sam --version
- You should see the version number of AWS SAM CLI
If you have problems, check the AWS SAM CLI troubleshooting guide for help.
Setting up your development environment
To test serverless apps locally with AWS SAM CLI, you need to set up your development environment. This section will show you how to set up AWS credentials, make a new SAM project, and understand the SAM template file.
Setting up AWS credentials
To use AWS services, you need to set up your AWS credentials. You can do this in two ways:
-
Create a
credentials
file:-
Make a new file named
credentials
in the~/.aws/
folder -
Add your AWS access key ID and secret access key like this:
[default] aws_access_key_id = YOUR_ACCESS_KEY_ID aws_secret_access_key = YOUR_SECRET_ACCESS_KEY
-
-
Use AWS CLI:
- Run
aws configure
in your terminal - Follow the steps to enter your AWS access key ID and secret access key
- Run
Creating a new SAM project
To make a new SAM project:
- Open your terminal
- Run
sam init
- Choose a project template when asked
- SAM will create a new folder with your project files
Understanding the SAM template file
The SAM template file (template.yaml
) defines your serverless app. It includes details about your Lambda functions, API Gateway, and other resources.
Here's a simple SAM template file:
AWSTemplateFormatVersion: '2010-09-09'
Transform: AWS::Serverless-2016-10-31
Resources:
HelloWorldFunction:
Type: AWS::Serverless::Function
Properties:
FunctionName:!Sub 'hello-world-${AWS::Region}'
Runtime: nodejs14.x
Handler: index.handler
CodeUri: hello-world/
This template defines a Lambda function called HelloWorldFunction
. It uses Node.js and is in the hello-world/
folder.
In the next part, we'll look at how to test your serverless app locally using SAM CLI.
sbb-itb-6210c22
Testing locally with SAM CLI
Testing serverless apps locally with AWS SAM CLI helps you find and fix problems before putting your app on AWS.
Running Lambda functions locally
To run a Lambda function on your computer:
- Go to your SAM project folder
- Use this command:
sam local invoke FunctionName
This runs the function and shows the result in your terminal.
To use test data:
sam local invoke FunctionName --event event.json
This runs the function with data from the event.json
file.
Testing API Gateway locally
To test API Gateway on your computer:
- Use this command:
sam local start-api
- Test your API with a tool like
curl
:
curl http://localhost:3000/hello
This sends a GET request to /hello
and shows the response.
Testing event-driven apps
To test event-driven apps:
- Make a sample event:
sam local generate-event s3 > event.json
- Use the event to test your function:
sam local invoke FunctionName --event event.json
Finding and fixing problems
SAM CLI helps you find and fix problems in your app:
Tool | Command | What it does |
---|---|---|
Debugging | sam local invoke FunctionName --debug |
Lets you step through your code |
Logs | sam local logs FunctionName |
Shows logs for your function |
Use these tools to check your app and make sure it works well before putting it on AWS.
Advanced SAM CLI features
Using custom runtimes
AWS SAM CLI lets you use custom runtimes for specific programming needs. A custom runtime is a package that includes the language runtime, libraries, and dependencies your app needs. With custom runtimes, you can:
- Use languages or frameworks not supported by AWS Lambda
- Make your app run faster with a custom-compiled runtime
- Use specific versions of languages or libraries not available in standard AWS Lambda
To create a custom runtime:
- Make a Docker image with your required language runtime, libraries, and dependencies
- Add a
runtime
section to yourtemplate.yaml
file that points to the custom runtime image - Use
sam build
to create a deployment package with the custom runtime
Here's an example of a runtime
section in template.yaml
:
AWSTemplateFormatVersion: '2010-09-09'
Transform: AWS::Serverless-2016-10-31
Resources:
MyFunction:
Type: AWS::Serverless::Function
Properties:
Handler: index.handler
Runtime: nodejs14.x
CodeUri: .
Environment:
Variables:
BUCKET_NAME: !Sub 'my-bucket-${AWS::Region}'
Events:
MyEvent:
Type: Api
Properties:
Path: '/my-path'
Method: get
CustomRuntime:
Type: AWS::EC2::Image
Properties:
Name: my-custom-runtime
Description: Custom Node.js 14.x runtime
BlockDeviceMappings:
- DeviceName: /dev/xvda
Ebs:
VolumeSize: 30
VolumeType: gp2
DeleteOnTermination: true
InstanceType: t2.micro
ImageLocation: my-custom-runtime-image
In this example, CustomRuntime
defines a custom Node.js 14.x runtime image used by MyFunction
.
Working with Step Functions
AWS SAM CLI works with AWS Step Functions to coordinate parts of distributed apps and microservices. Step Functions let you:
- Define a state machine to control your app components
- Use a visual tool to design and fix your state machine
- Work with other AWS services like Lambda, S3, and DynamoDB
To use Step Functions with AWS SAM CLI:
- Add a
StepFunction
resource to yourtemplate.yaml
file - Use
sam build
to make a deployment package with the Step Functions state machine - Use
sam deploy
to put your app on AWS, creating the state machine and setting up roles and permissions
Here's an example of a StepFunction
resource in template.yaml
:
AWSTemplateFormatVersion: '2010-09-09'
Transform: AWS::Serverless-2016-10-31
Resources:
MyStepFunction:
Type: AWS::StepFunctions::StateMachine
Properties:
DefinitionString: !Sub |
{
"StartAt": "FirstState",
"States": {
"FirstState": {
"Type": "Task",
"Resource": "${MyFunction}",
"Next": "SecondState"
},
"SecondState": {
"Type": "Task",
"Resource": "${MyOtherFunction}",
"End": true
}
}
}
RoleArn: !GetAtt 'MyExecutionRole.Arn'
MyFunction:
Type: AWS::Serverless::Function
Properties:
Handler: index.handler
Runtime: nodejs14.x
CodeUri: .
Environment:
Variables:
BUCKET_NAME: !Sub 'my-bucket-${AWS::Region}'
Events:
MyEvent:
Type: Api
Properties:
Path: '/my-path'
Method: get
MyOtherFunction:
Type: AWS::Serverless::Function
Properties:
Handler: index.handler
Runtime: nodejs14.x
CodeUri: .
Environment:
Variables:
BUCKET_NAME: !Sub 'my-bucket-${AWS::Region}'
Events:
MyOtherEvent:
Type: Api
Properties:
Path: '/my-other-path'
Method: get
This example shows MyStepFunction
controlling two Lambda functions, MyFunction
and MyOtherFunction
.
Using Lambda layers
AWS SAM CLI supports Lambda layers, which let you package app dependencies in a separate layer for reuse across functions. Lambda layers help you:
- Share dependencies across multiple functions
- Provide the same dependencies for multiple functions
- Make your deployment package smaller by excluding shared dependencies
To use Lambda layers with AWS SAM CLI:
- Add a
LayerVersion
resource to yourtemplate.yaml
file - Use
sam build
to create a deployment package with the layer - Use
sam deploy
to put your app on AWS, creating the layer and setting up roles and permissions
Here's an example of a LayerVersion
resource in template.yaml
:
AWSTemplateFormatVersion: '2010-09-09'
Transform: AWS::Serverless-2016-10-31
Resources:
MyLayer:
Type: AWS::Serverless::LayerVersion
Properties:
LayerName: !Sub 'my-layer-${AWS::Region}'
Description: My layer
Content:
S3Bucket: !Sub 'my-bucket-${AWS::Region}'
S3Key: my-layer.zip
CompatibleRuntimes:
- nodejs14.x
LicenseInfo: MIT
MyFunction:
Type: AWS::Serverless::Function
Properties:
Handler: index.handler
Runtime: nodejs14.x
CodeUri: .
Environment:
Variables:
BUCKET_NAME: !Sub 'my-bucket-${AWS::Region}'
Events:
MyEvent:
Type: Api
Properties:
Path: '/my-path'
Method: get
Layers:
- !Ref MyLayer
In this example, MyLayer
is a layer with shared dependencies, and MyFunction
uses this layer for its dependencies.
Best practices for local testing
Organizing your code for testing
When testing serverless apps locally, organize your code well. This helps you test and fix problems easily. Here's what to do:
Practice | Description |
---|---|
Split code into modules | Break code into small, separate parts |
Use clear names | Name files, functions, and variables clearly |
Make code reusable | Write code that can be used in different parts of your app |
These practices make your code easier to test, fix, and use.
Handling environment variables
Environment variables let you change how your app works without changing the code. Here's how to use them when testing locally:
Practice | Description |
---|---|
Use a .env file |
Keep environment variables in one file |
Name variables clearly | Use names that show what each variable does |
Keep secrets safe | Store sensitive info like API keys separately |
These practices help you manage environment variables well and keep your app safe.
Simulating AWS services
Testing how your app works with AWS services is important. Here's how to do it:
Practice | Description |
---|---|
Use AWS SAM CLI | Test with API Gateway and Lambda on your computer |
Use mocking tools | Test with other AWS services not in SAM CLI |
Check for problems | Test what happens when things go wrong |
These practices help you make sure your app works well with AWS services before you put it online.
Common issues and solutions
Fixing permission problems
When testing serverless apps locally with AWS SAM CLI, you might face permission issues. Here's how to fix them:
Problem | Solution |
---|---|
IAM permissions | Check your IAM user or role has the right permissions |
AWS credentials | Run aws sts get-caller-identity to verify your credentials |
AWS region | Use the correct region in samconfig.toml or with --region option |
Resolving dependency conflicts
To manage library dependencies:
Method | Description |
---|---|
Virtual environment | Use virtualenv or conda to isolate project dependencies |
requirements.txt |
List all needed dependencies in this file |
Dependency manager | Use pip or npm to handle dependencies and versions |
Solving Docker-related issues
Common Docker problems and fixes:
Issue | Solution |
---|---|
Docker not installed | Download and install from Docker website |
Docker not running | Check status with docker ps command |
Permission issues | Add user to Docker group: sudo usermod -aG docker $USER |
Missing Docker image | Pull image from Docker Hub: docker pull <image-name> |
These tips should help you tackle common problems when using AWS SAM CLI for local testing.
Conclusion
Key points to remember
We covered how to test serverless apps locally using AWS SAM CLI. Here's what we learned:
Topic | Details |
---|---|
Setup | AWS account, AWS CLI, Docker |
AWS SAM CLI basics | Main features, local testing benefits |
Development environment | AWS credentials, new SAM project |
Local testing methods | Lambda functions, API Gateway, event-driven apps |
The value of local testing
Testing locally is important for serverless apps. It helps developers:
- Find and fix problems early
- Test code before putting it on AWS
- Save time and money
AWS SAM CLI makes local testing easier by:
- Copying the AWS setup on your computer
- Letting you test and fix code locally
To sum up, local testing is key for making good serverless apps. AWS SAM CLI is a helpful tool for this. By following the steps in this article, you can set up local testing and start improving your serverless apps today.
Best Practices for Local Testing
Practice | Description |
---|---|
Test thoroughly | Check everything before putting it on AWS |
Use AWS SAM CLI | Copy AWS on your computer |
Set up correctly | Get AWS login info and make a new SAM project |
Test all parts | Check Lambda, API Gateway, and event-driven apps |
Use debugging tools | Fix problems with AWS SAM CLI |
FAQs
How do I test a serverless application locally?
You can test a serverless application on your computer using AWS SAM CLI. Here's how:
1. Install AWS SAM CLI
- Go to the AWS website
- Follow the steps to install AWS SAM CLI
2. Make a SAM project
- Use AWS SAM CLI to create a new project
- This gives you a basic setup for your app
3. Write your app code
- Create Lambda functions
- Set up API Gateway
- Add any other needed code
4. Test your app
- Use
sam local start-api
to run a local API Gateway - Use
sam local invoke
to run Lambda functions
By doing this, you can check your app before putting it on AWS.
Why test locally? | How it helps |
---|---|
Quick feedback | See if your code works right away |
Save money | Fewer uploads to AWS means lower costs |
Better code | Find and fix problems early |
Testing your app locally with AWS SAM CLI is a key part of making serverless apps. It helps you spot issues quickly and cheaply, leading to better apps overall.